Consider a few cases for working with the Flag module.
How to display a flag in any place of your template
Consider how to display a flag reference at a specific place in your own node template. Let it be an Article. Create a file node--article.tpl.php in the your theme folder. The contents of the file are the same as standard node template node.tpl.php.
Let's say we want to display a flag under the title. I add a stub to the template code:
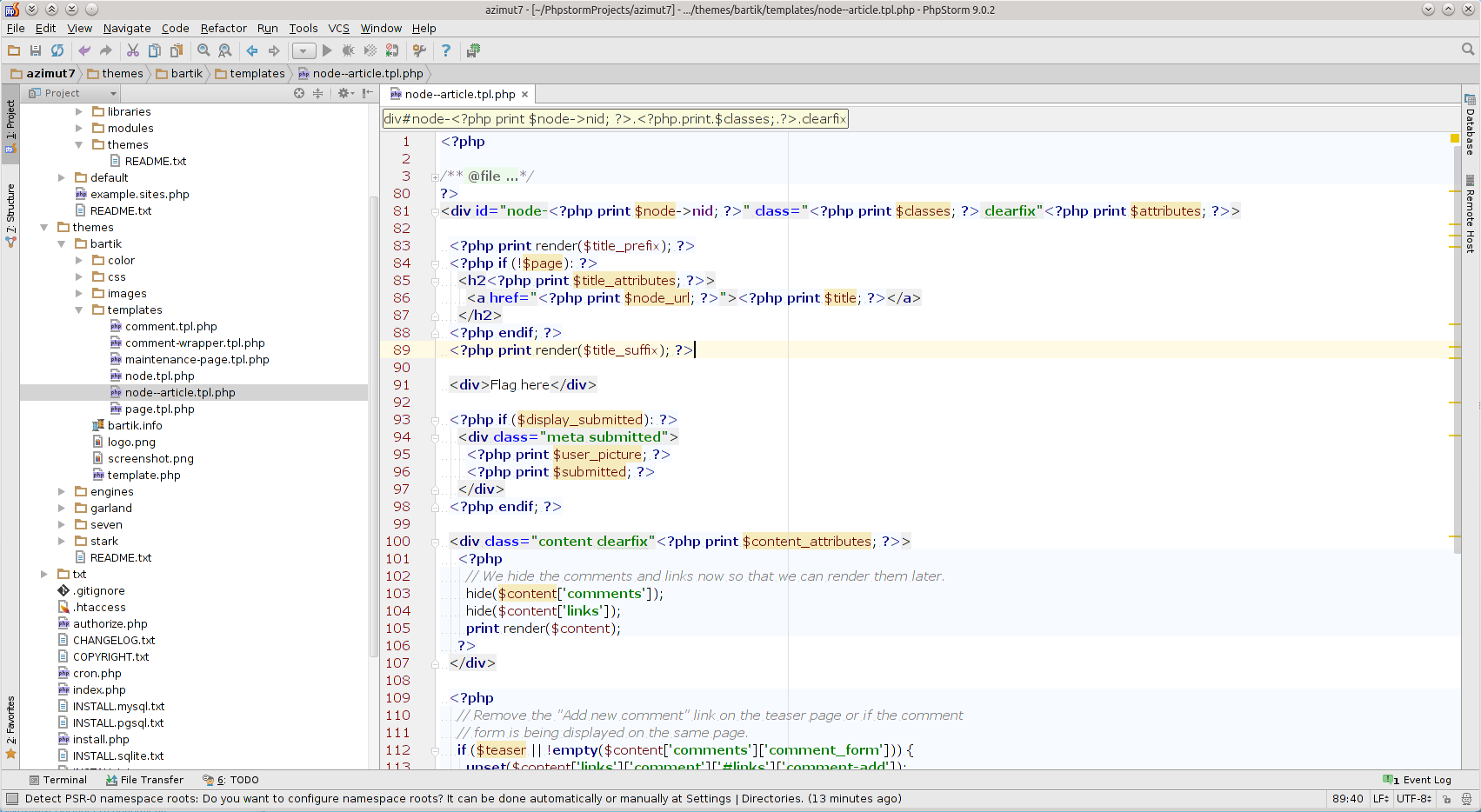
If your template works, you will immediately see it in the browser. If not, clear the Drupal cache at admin/config/development/performance.
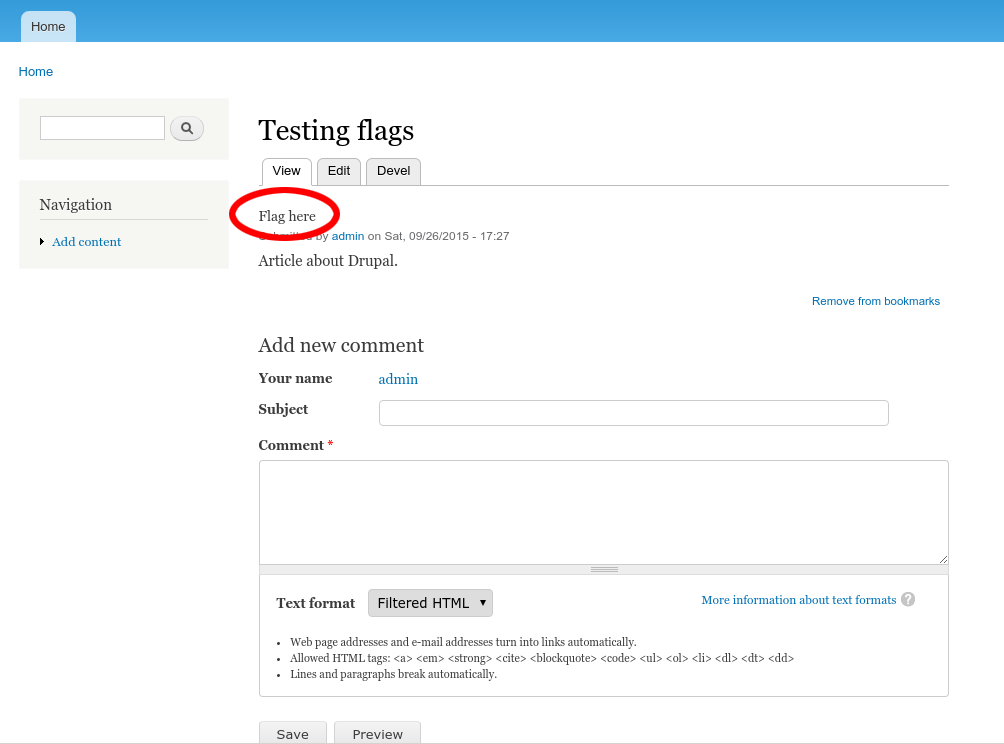
To display flag links, you can use the following code:
$flag = render($content['links']['flag']);
print $flag;
However, there may be a problem, if several flags are attached to the node, you will see all of them, and not the one you need. Let's check the output of all flags.
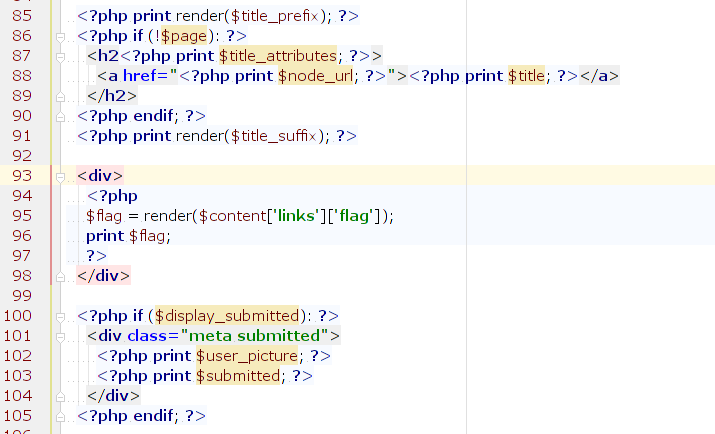
I got two different flags: bookmarks and one test flag.
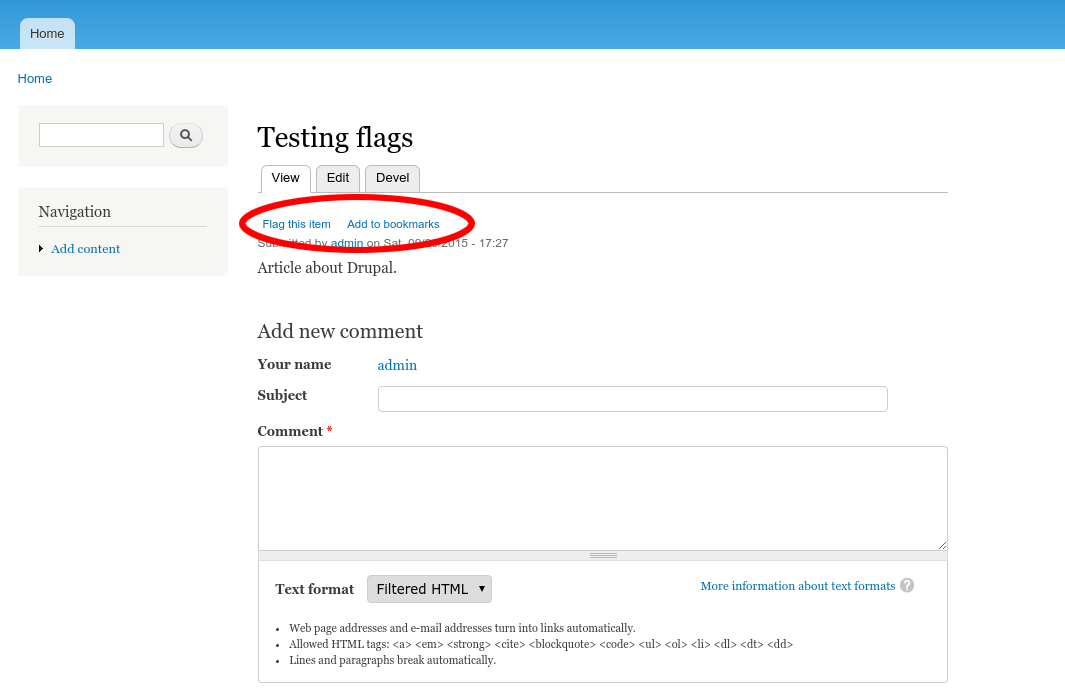
How to display only one flag. To do this, display the value of the $content['links']['flag'] variable on the screen. If you have a Devel module, the output will be:
dpm($content['links']['flag']);
This code can be inserted at the beginning of the node--article.tpl.php, and you will see the following information on the screen:
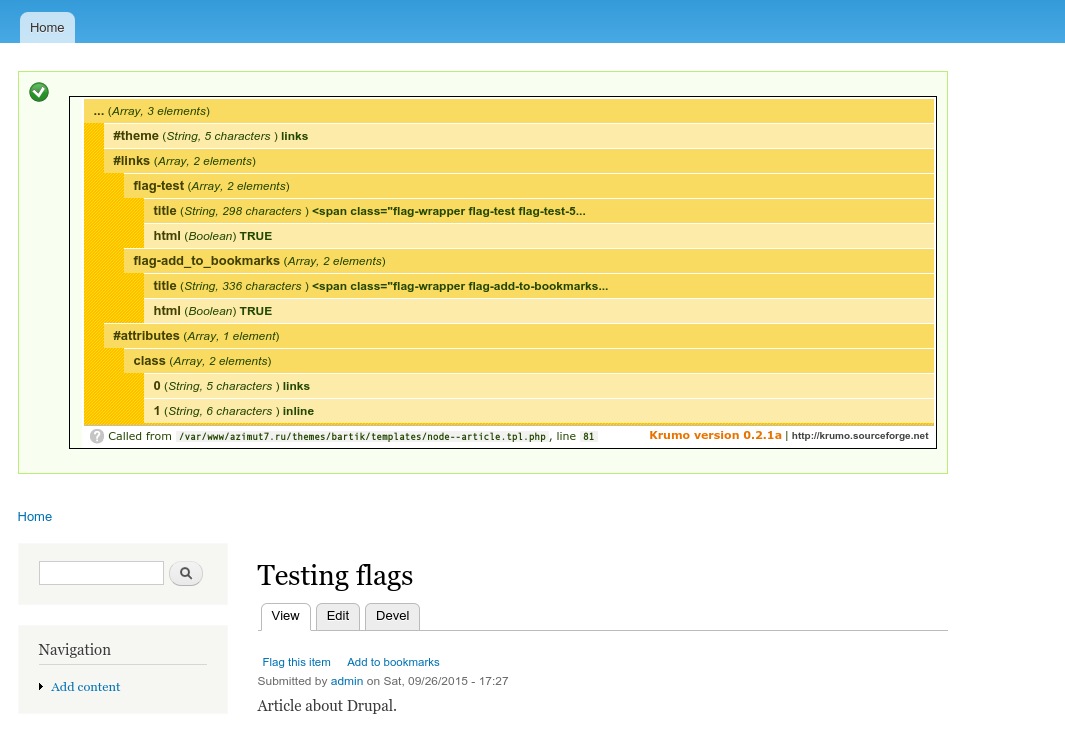
The array element with the #links key will contain all the flags that are attached to this type of material. Let's remove all unnecessary flags:
$my_flag = 'add_to_bookmarks'; // Our flag
$all_flags = $content['links']['flag'];
foreach($all_flags['#links'] as $flag_name => $flag_value) {
if ($flag_name != 'flag-' . $my_flag) {
// Remove other flags
unset($all_flags['#links'][$flag_name]);
}
}
// Output our flag
$one_flag = render($all_flags);
print $one_flag;
Place this code where you want to display the flag.
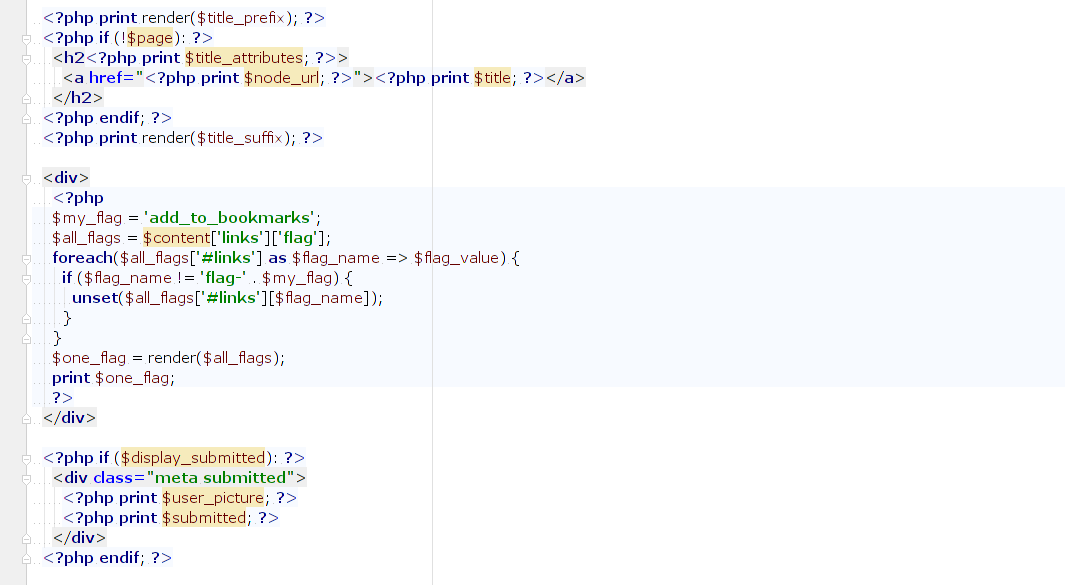
If you did everything correctly, you will see only the flag you need on the page.
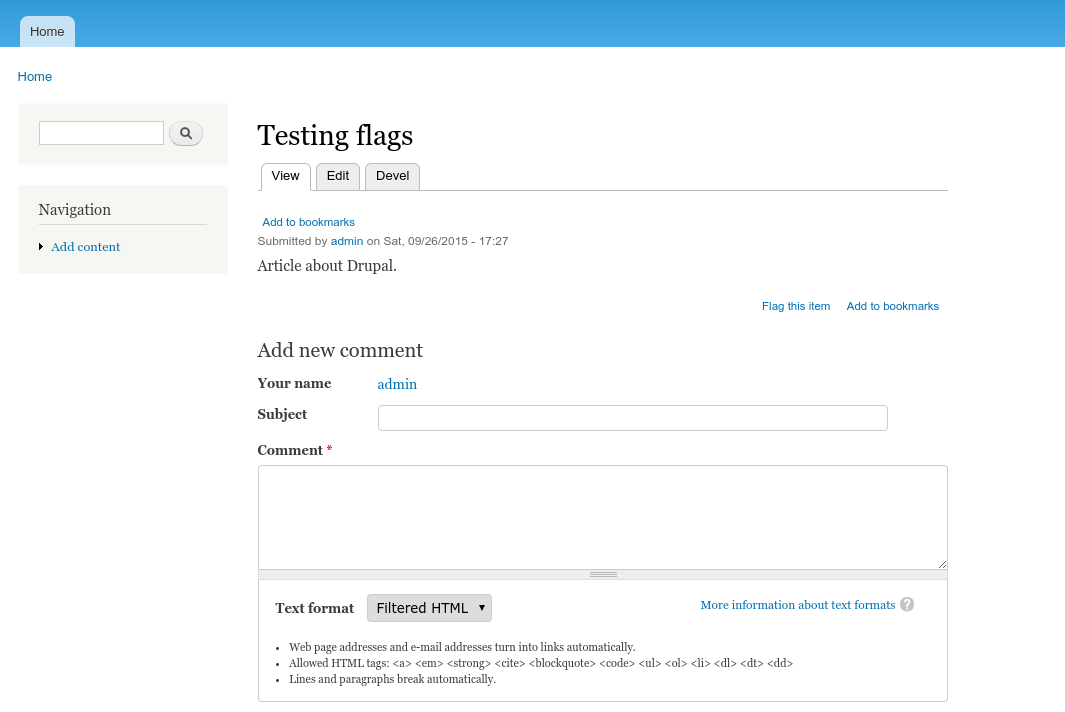
How programmatically flag the content
You need your own module. Let's create a module with a single function that we will call through Devel.
Create azimut7.info:
name = Azimut7
description = Helper module.
core = 7.x
version = "7.x-1.0"
core = "7.x"
project = "azimut7"
datestamp = "1398963366"
And the module file azimut7.module. The file will contain only one function, which will flag the specific material.
function azimut7_flag_prog($flag_name, $nid) {
}
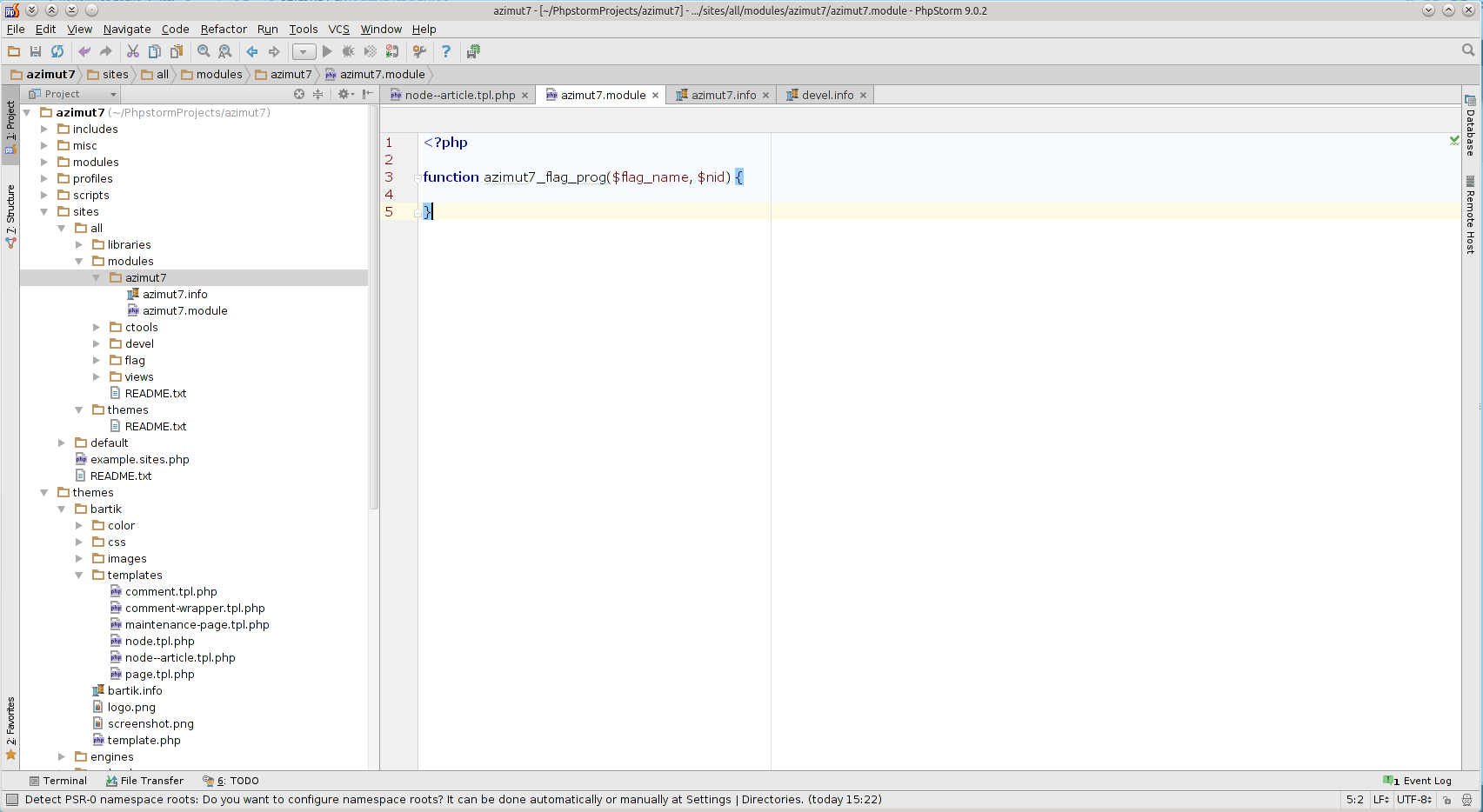
Turn on your new module. After that, the function azimut7_flag_prog will be available for the call.
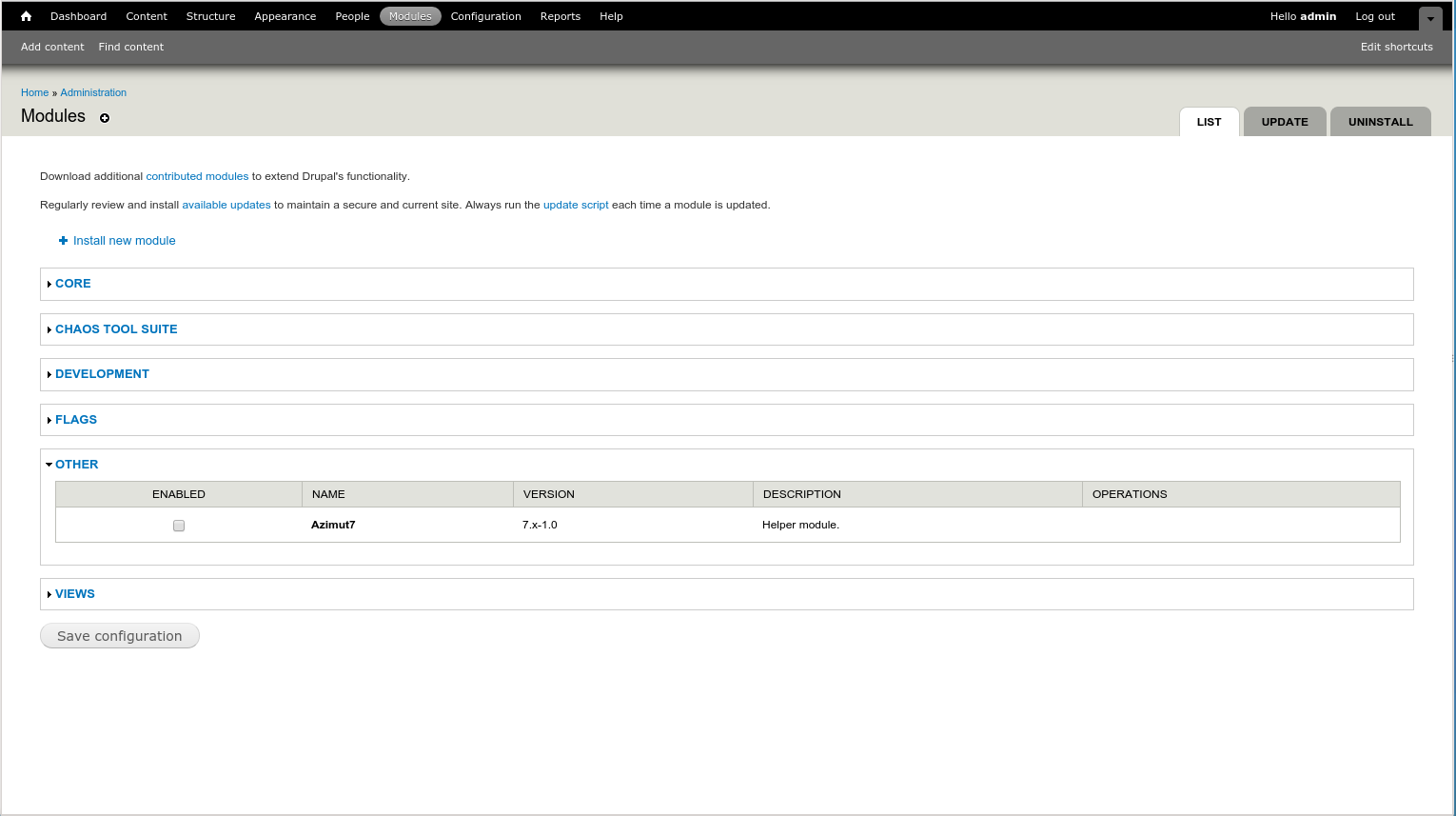
Now to check the functionality of the function, go to devel/php and write in the input field::
azimut7_flag_prog(1,2);
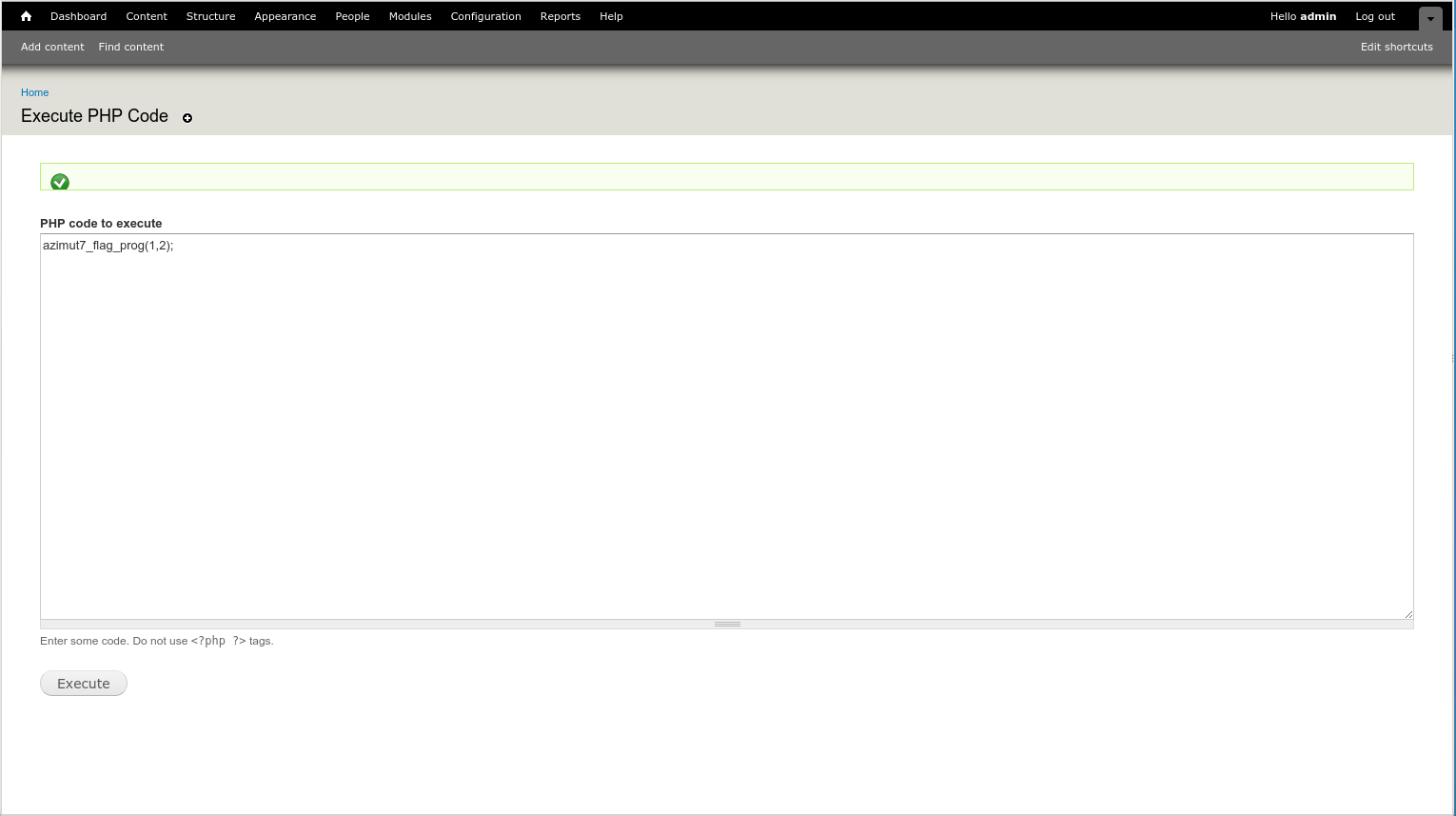
For the test, I passed 1 and 2 to the function. If there are no errors, then the module is correctly turned on and working.
Write this code in azimut7_flag_prog function:
// Current user
global $user;
// Load the necessary flag
$flag = flag_get_flag($flag_name);
// Flag the necessary material on behalf of the current user
$flag->flag('flag', $nid, user_load($user->uid));
Now try running this function in the Devel module.
At devel/php we write:
// 1 - this is node ID you need
azimut7_flag_prog('add_to_bookmarks',1);
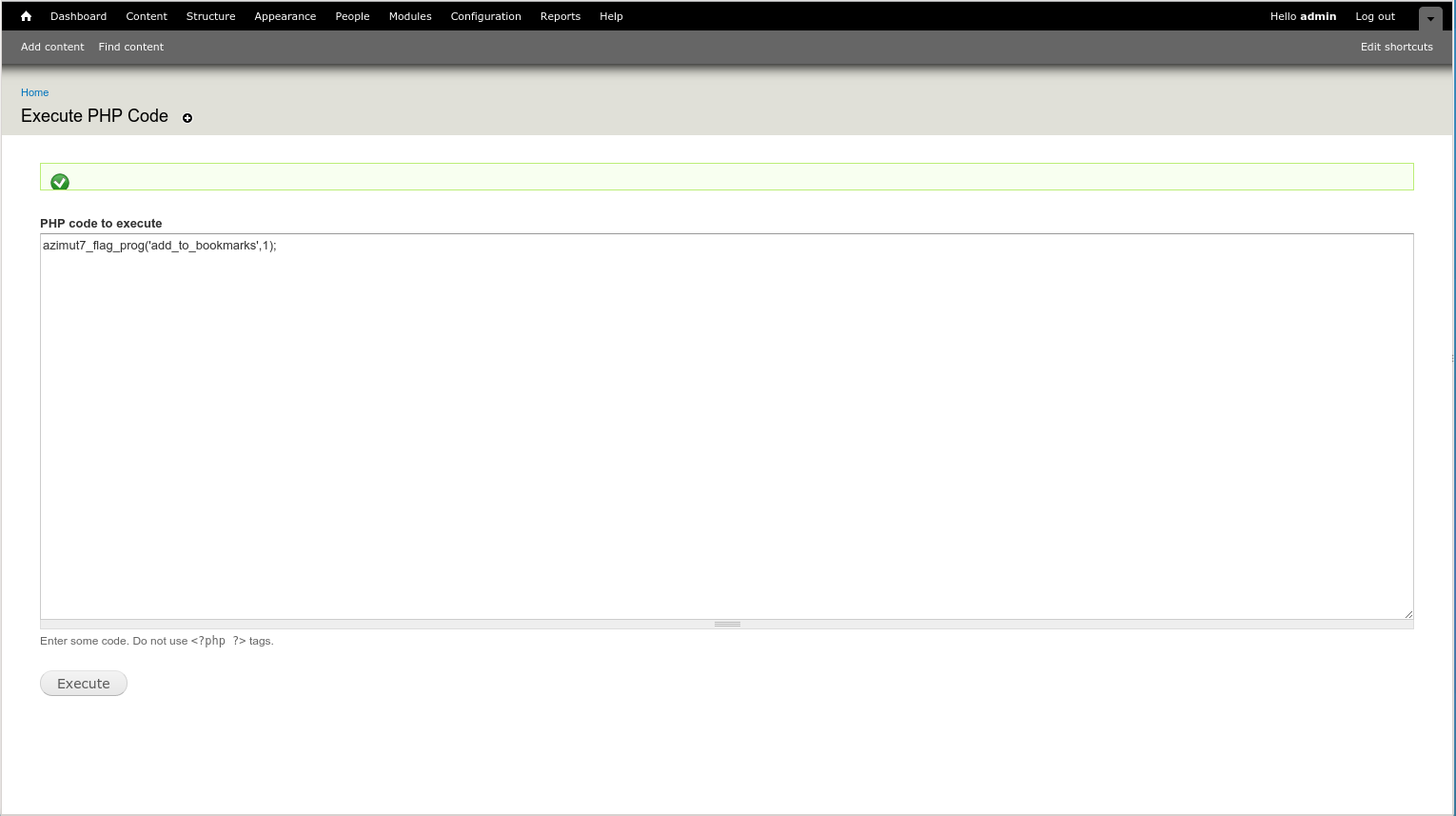
After that, you can check the material, the flag will stand. If you need to remove the flag, then you should pass the string unflag to the flag method as the first argument.
Let's just put it in the arguments of our function to make it clearer.
function azimut7_flag_prog($flag_name, $nid, $action = 'flag') {
global $user;
$flag = flag_get_flag($flag_name);
$flag->flag($action, $nid, user_load($user->uid));
}
Set the flag programmatically:
azimut7_flag_prog('add_to_bookmarks', 1,'flag');
// or
azimut7_flag_prog('add_to_bookmarks', 1);
Unset the flag:
azimut7_flag_prog('add_to_bookmarks', 1,'unflag');
In the first case, the third argument can be omitted because the default value is set in azimut7_flag_prog declaration.