With the Rules module, you can respond to various events on the website. For example add some actions that are performed after starting cron, creating an article, adding a comment, user registration, and many other events.
The functionality of the Rules gives the ability to create events from a variety of conditions. And you can attach various actions to these events. For example, sending e-mail, running a script, and so on.
Module install
Install the module via Drush:
drush dl rules && drush en -y rules_admin
We need to enable Rules and Rules Admin
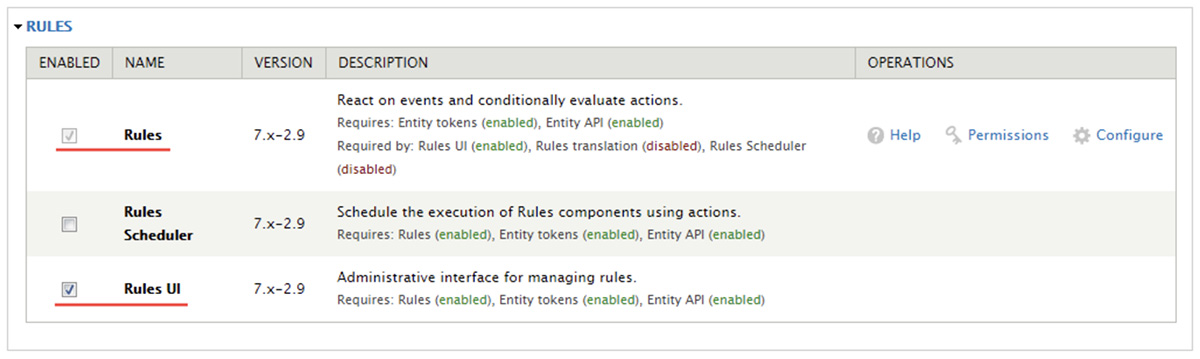
The Rules module depends on the Entity module, it must also be downloaded and enabled.
The rules page is located at admin/config/workflow/rules. There you will see already created rules and you will be able to add new ones.
Own event
Suppose we have a task to create an event in the Rules module when a user adds more than three materials in the last 24 hours.
To create your event, you need to create a custom module. Let's name it azimut7_rules. It will contain elements for the Rules module and must be dependent on it.
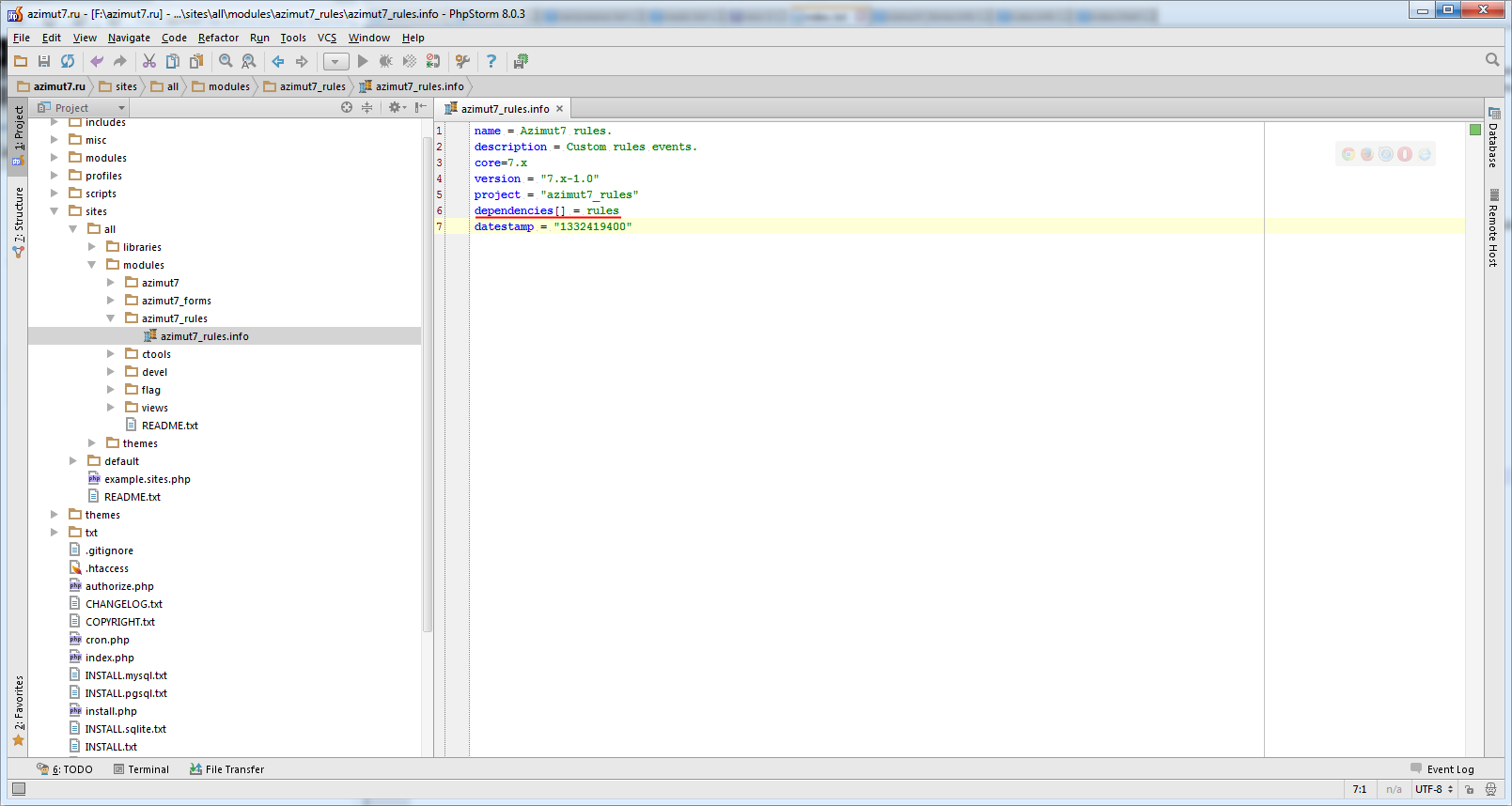
File azimut7_rules.info:
name = Azimut7 rules.
description = Custom rules events.
core=7.x
version = "7.x-1.0"
project = "azimut7_rules"
dependencies[] = rules
Let's "fix" this event in the module file. To do this, we need to cling to the event of adding new material and count in it how much the user has created in the last 24 hours. Add a hook and see if it works.
azimut7_rules.module:
function azimut7_rules_node_insert($node) {
dpm($node);
}
Do not forget to enable the module.
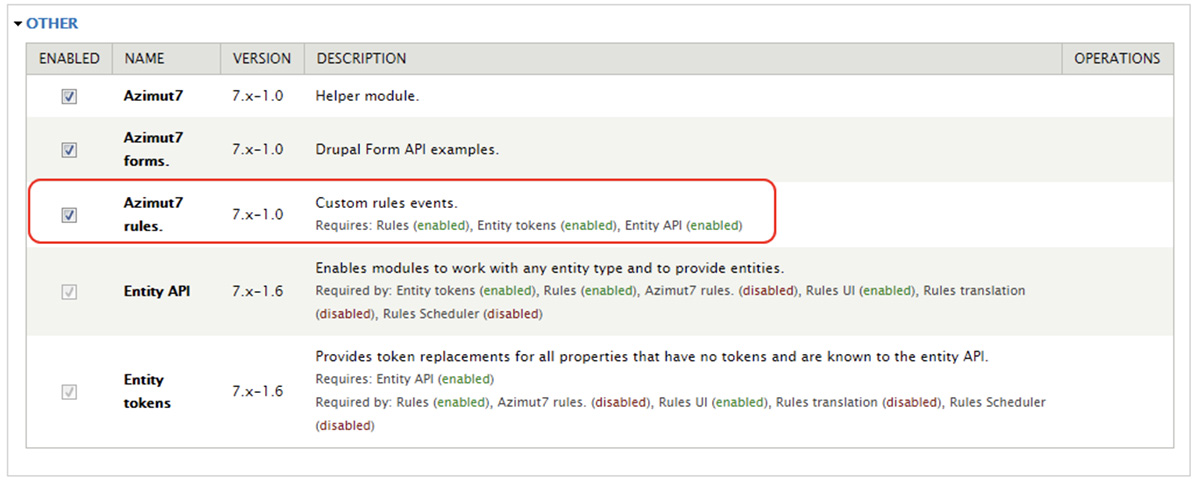
I tried to create the material and saw the output of my debug message. This suggests that the hook is working. Let's write the required check.
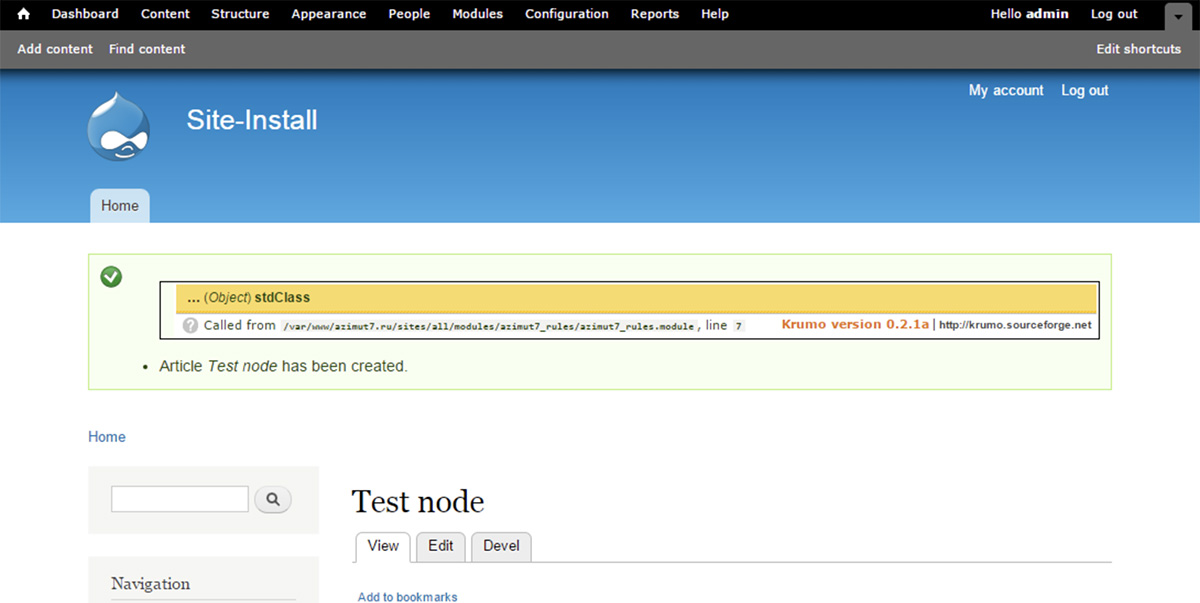
Write a function:
function azimut7_rules_node_insert($node) {
global $user;
$time = strtotime('-1 day');
$node_count = db_select('node', 'n')
->fields('n', array('nid'))
->condition('n.uid', $user->uid)
->condition('n.created', $time, '>')
->execute()
->rowCount();
dpm($node_count);
}
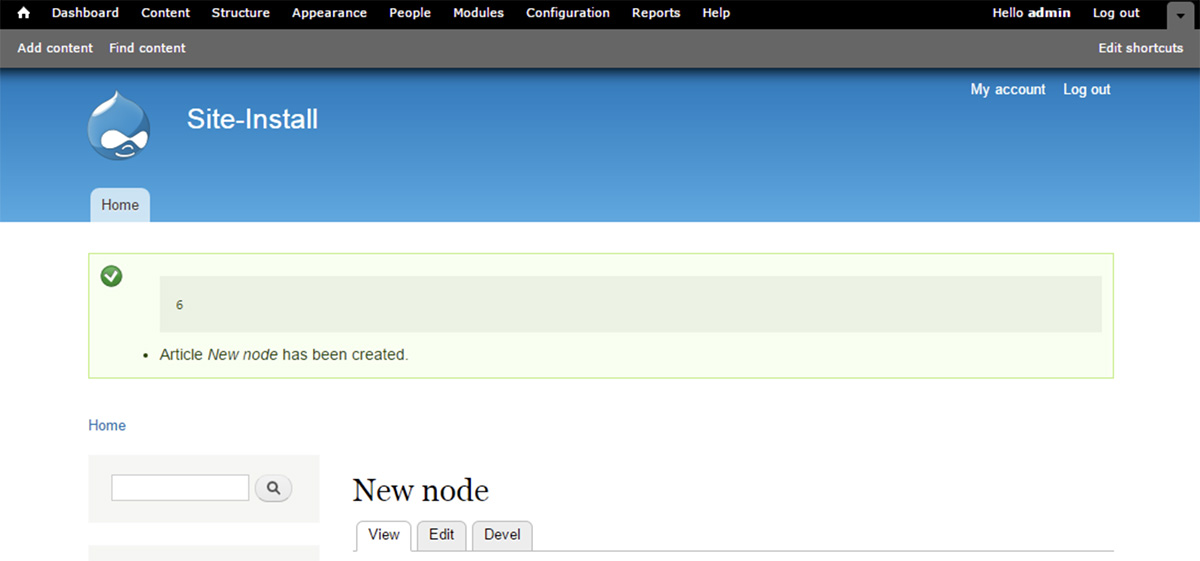
With this query, we will find the amount of content created by the current user in the last 24 hours. The created material is also included in this figure. Try to create a material and you will see the value you are looking for.
Now you need to create the event itself. To do this, create a file named azimut7_rules.rules.inc and announce event in it:
function azimut7_rules_rules_event_info() {
$items = array(
'azimut7_rules_created_node_count' => array(
'label' => t('Count of last user nodes'),
'category' => 'node',
),
);
return $items;
}
In order for Drupal to know about this event, you need to add info about this file:
name = Azimut7 rules.
description = Custom rules events.
core=7.x
version = "7.x-1.0"
files[] = azimut7_rules.rules.inc
project = "azimut7_rules"
dependencies[] = rules
Do not forget to clear the site cache.
Now we can call (rules_invoke_event) the created event from our first hook:
function azimut7_rules_node_insert($node) {
global $user;
$time = strtotime('-1 day');
$node_count = db_select('node', 'n')
->fields('n', array('nid'))
->condition('n.uid', $user->uid)
->condition('n.created', $time, '>')
->execute()
->rowCount();
if ($node_count >= 3) {
rules_invoke_event('azimut7_rules_created_node_count');
}
}
We have finished writing the code and now we can create a rule.
Open admin/config/workflow/rules/reaction/add. On the page will be our event. Choose it:
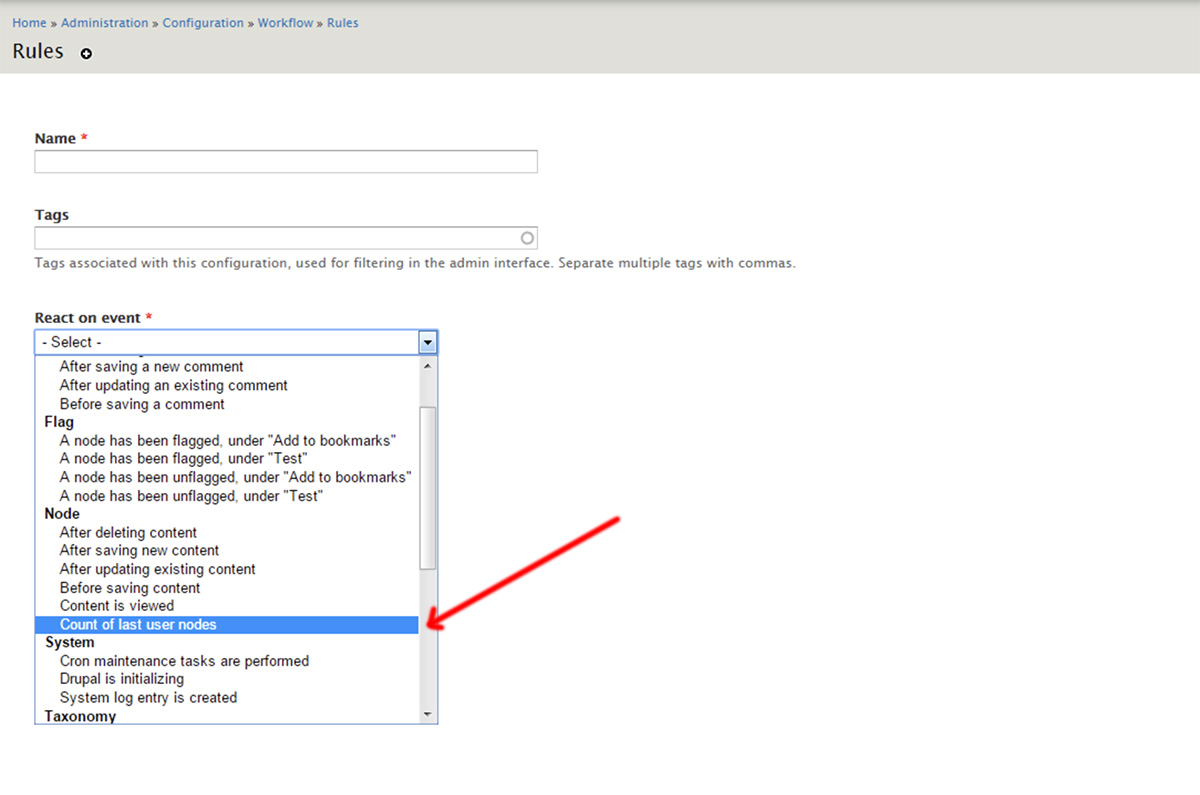
You need to fill in the field with the name of the rule, you can add a tag:
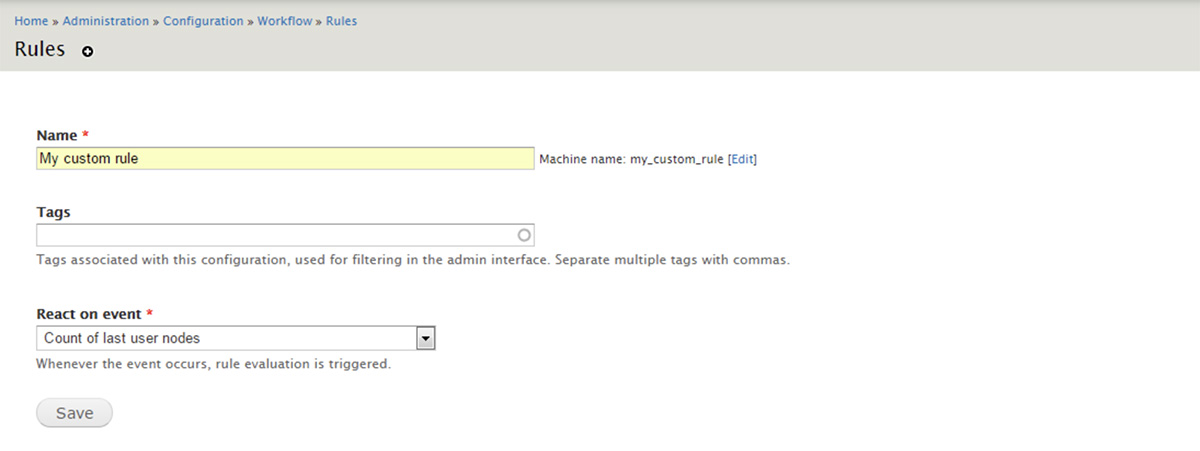
Now let's add an action for this event, for example, display a message. Click Add action and select the message:
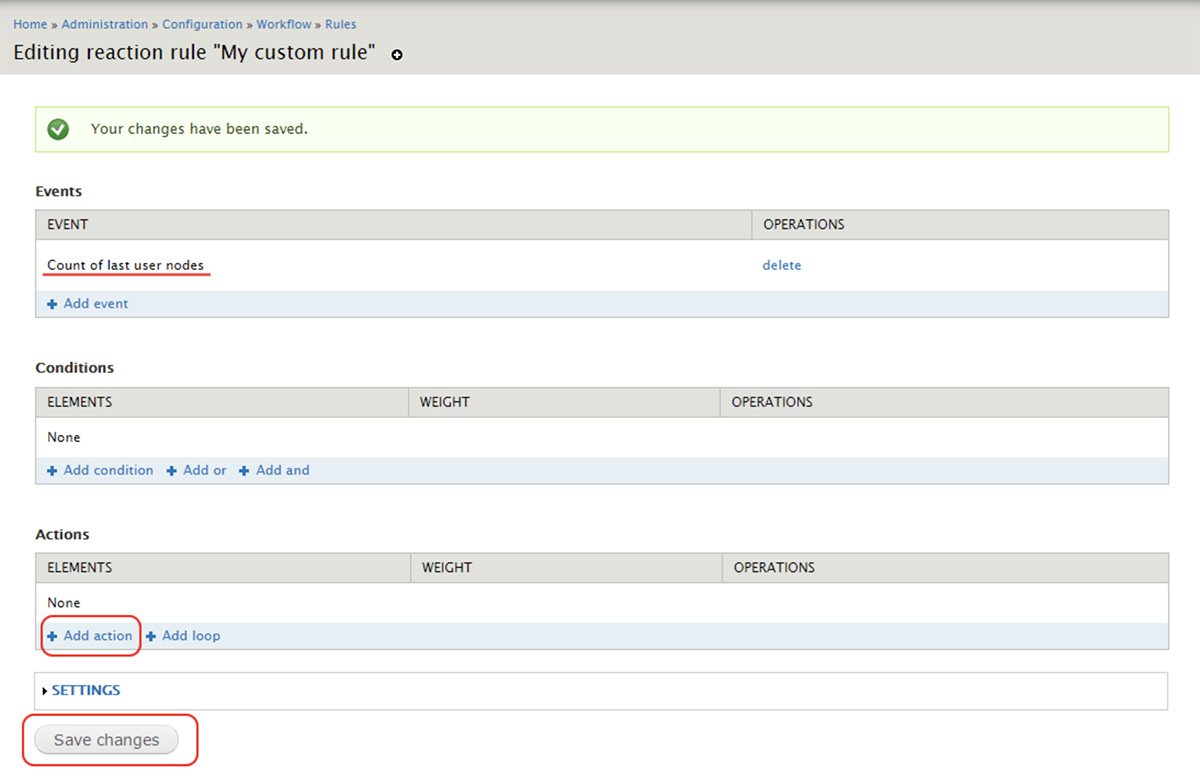
We write the text of the message and click Save.
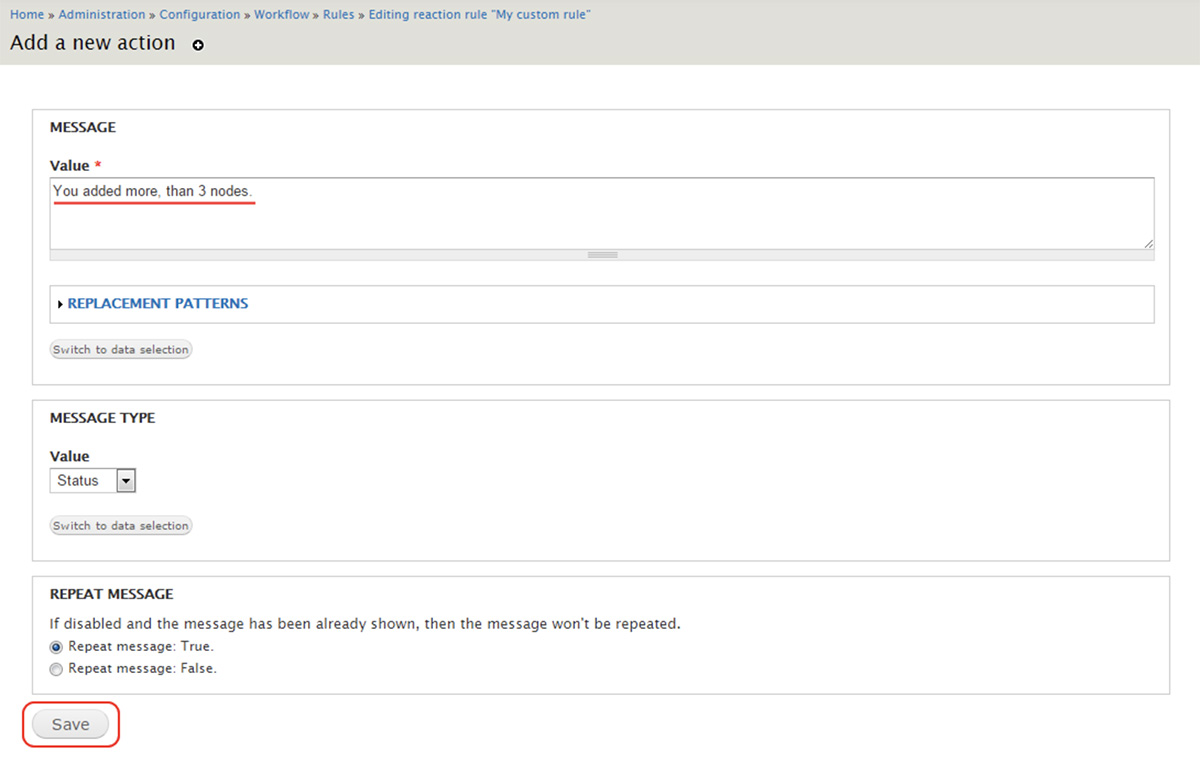
The rule is now ready. You can go to the list of all rules and see our rule:
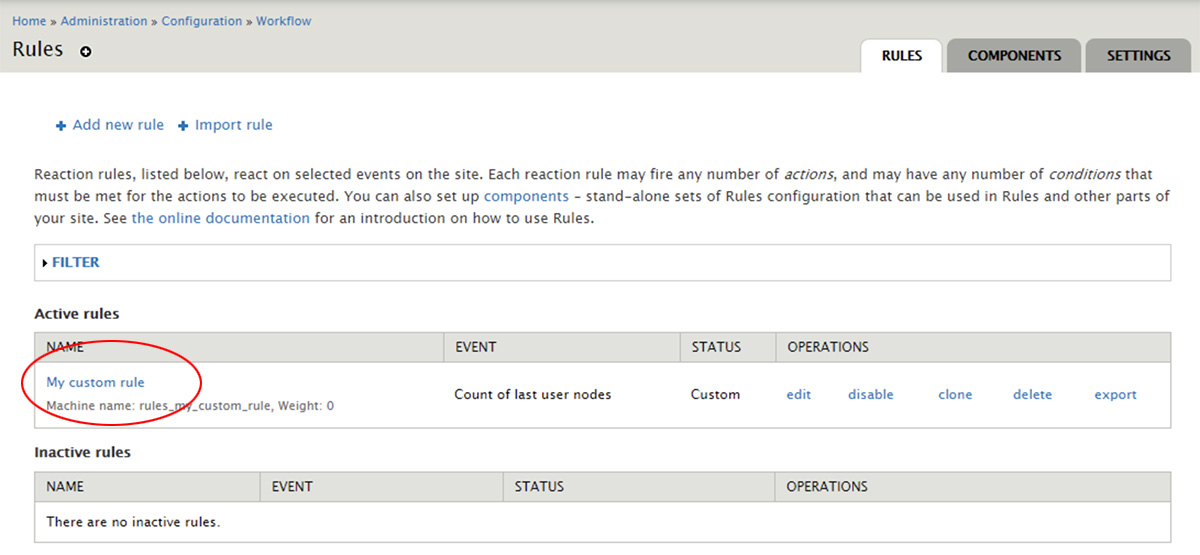
Create nodes and check our rule. I have created more than three nodes and see my message:
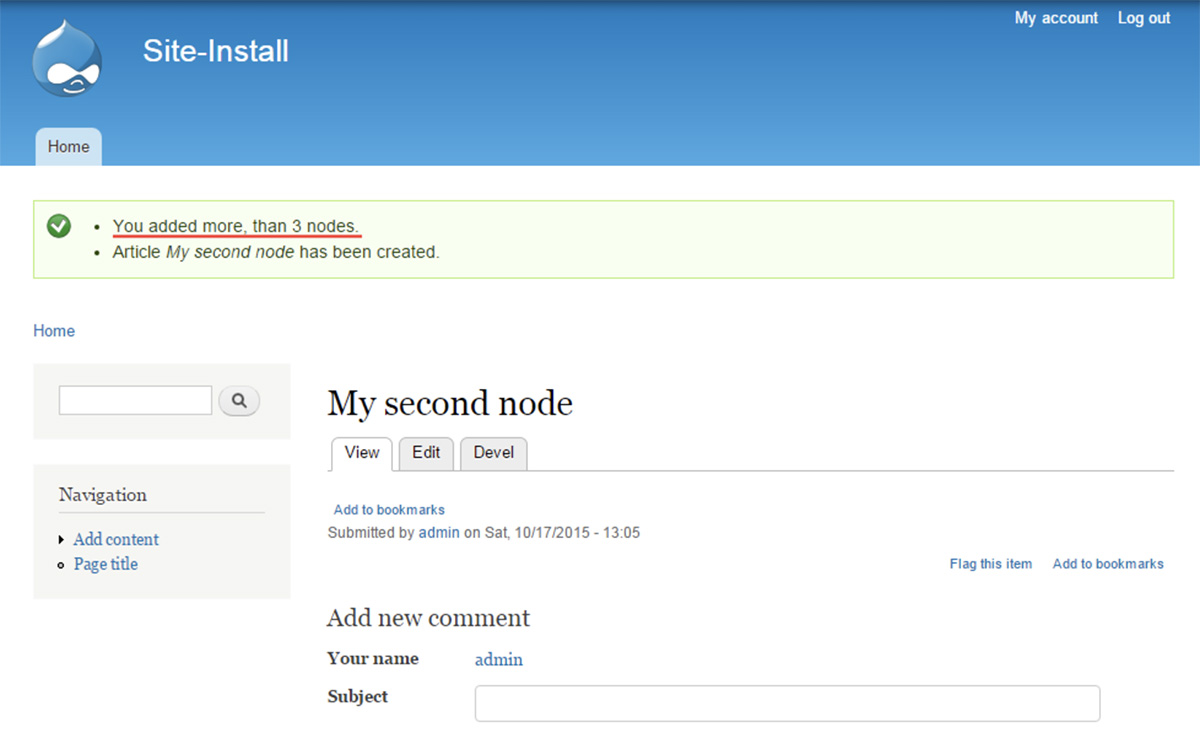